Build fully functional accessible web applications faster than ever – Mantine includes more than 100 customizable components and 40 hooks to cover you in any situation
Free and open source
All packages have MIT license, you can use Mantine in any project
TypeScript based
Build type safe applications, all components and hooks export types
Use anywhere
Mantine supports all modern frameworks: Next.js, Remix, etc.
100+ components
Every input can have description...
...and error
Dark color scheme
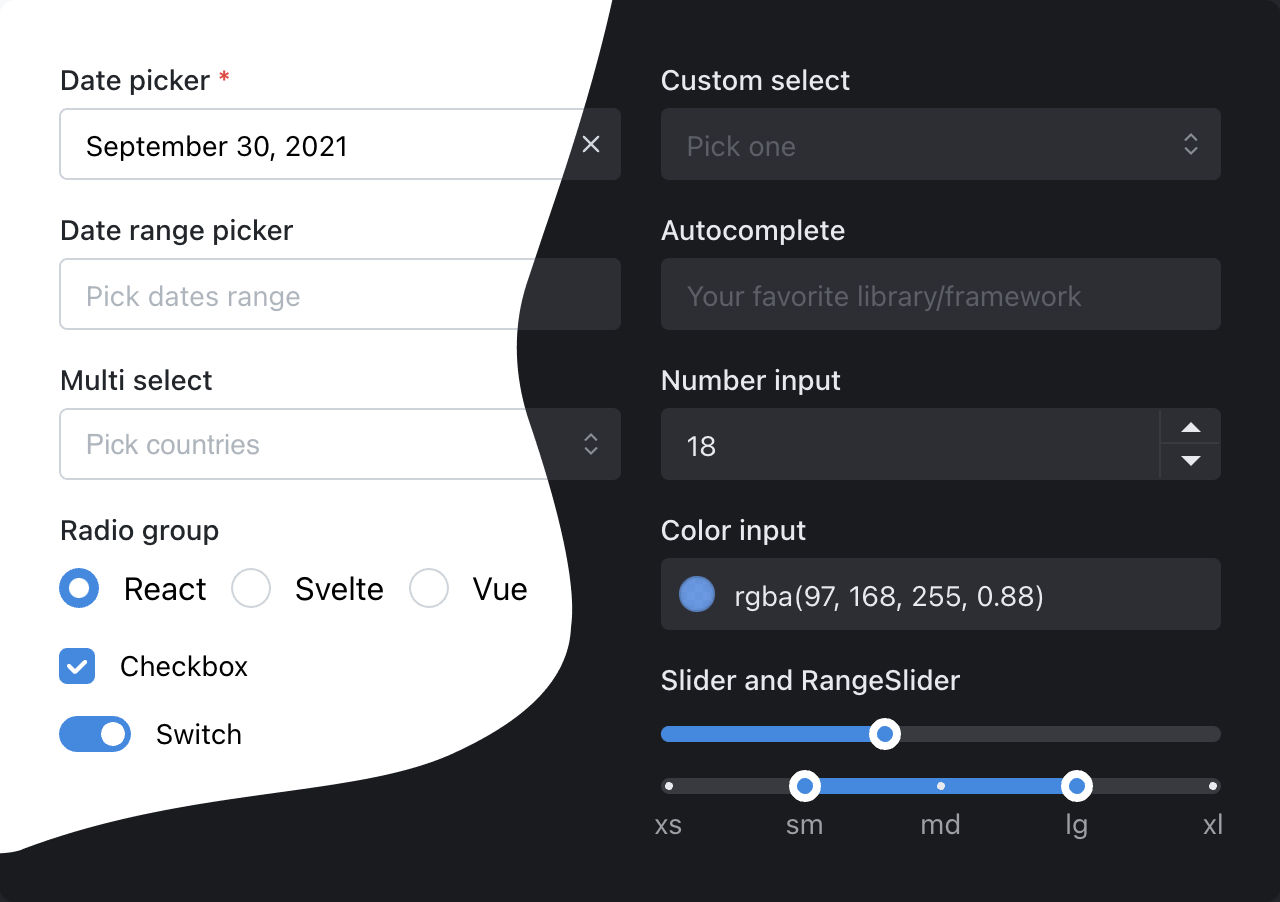
Add dark theme to your application with just a few lines of code – Mantine exports global styles both for light and dark theme, all components support dark theme out of the box.
Customize components
Every Mantine component supports visual customizations with props – you can quickly prototype and experiment by just modifying component props:
New branch
You've created new branch fix-notifications from master
2 hours ago
Commits
You've pushed 23 commits to fix-notifications branch
52 minutes ago
Pull request
You've submitted a pull request Fix incorrect notification message (#187)
34 minutes ago
Code review
Robert Gluesticker left a code review on your pull request
12 minutes ago
Color
Radius
xs
sm
md
lg
xl
Align
Styles overriding
Each Mantine component supports styles overriding for every internal element inside with classes or inline styles. This feature alongside other customization options allows you to implement any visual modifications to components and adapt them to fit almost any design requirements.
Default slider styles
20%
50%
80%
Find elements that you need to change in styles API table
Name | Description |
---|---|
root | Root element |
track | Track element, contains all other elements |
bar | Filled part of the track |
thumb | Main control |
dragging | Styles added to thumb while dragging |
label | Label element, displayed above thumb |
markWrapper | Wrapper around mark, contains mark and mark label |
mark | Mark displayed on the track |
markFilled | Styles added to mark when it is located in filled area |
markLabel | Mark label, displayed below track |
Flexible theming
Extend default theme with any amount of additional colors, replace shadows, radius, spacing, fonts and many other properties to match your design requirements.
Bright pink badge
Based on emotion
Mantine is based on emotion 👩🎤, take advantage of core emotion features: auto vendor-prefixing, critical css extraction during server side rendering, lazy evaluation, dynamic theming, type safe styles with TypeScript and more.
Add inline styles to any component with sx prop:
For more complex styles use createStyles function to separate styles from markup:
Subscribe to theme object in sx prop or createStyles function to use theme tokens in component styles:
You can use theme functions anywhere where Mantine theme is available:
Add context styles with MantineProvider that will be applied to every component:
Use styled components syntax with Mantine theme and @emotion/styled:
Hooks library
Resize textarea by dragging its right bottom corner
Width: 0px, height: 0px
Build even faster with Mantine UI
120+ responsive components
built with Mantine
Build your next website even faster with premade responsive components designed and built by Mantine maintainers and community. All components are free forever for everyone.
Ready to get started?
Mantine works in all modern environments – get started instantly with Next.js, Gatsby.js, create-react-app, Vite or Remix by following getting started guide: